576. Out of Boundary Paths
1. Question
There is an m x n
grid with a ball. The ball is initially at the position [startRow, startColumn]
. You are allowed to move the ball to one of the four adjacent cells in the grid (possibly out of the grid crossing the grid boundary). You can apply at most maxMove moves to the ball.
Given the five integers m
, n
, maxMove
, startRow
, startColumn
, return the number of paths to move the ball out of the grid boundary. Since the answer can be very large, return it modulo 109 + 7.
2. Examples
Example 1:
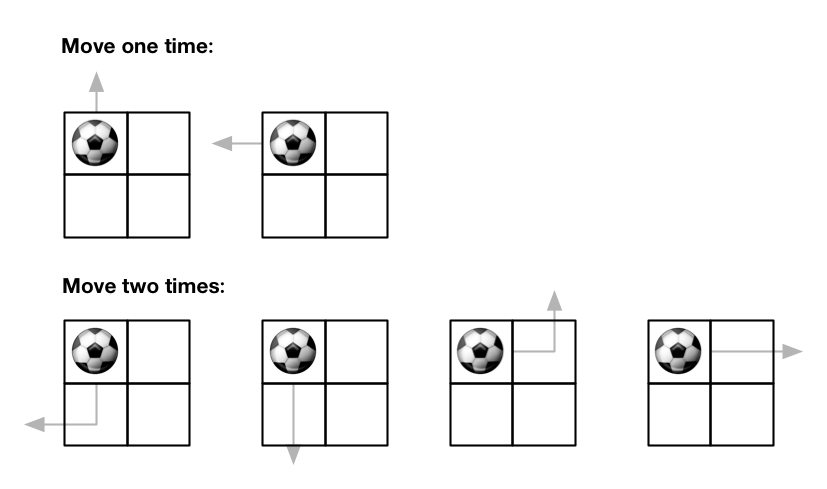
Input: m = 2, n = 2, maxMove = 2, startRow = 0, startColumn = 0
Output: 6
Example 2:
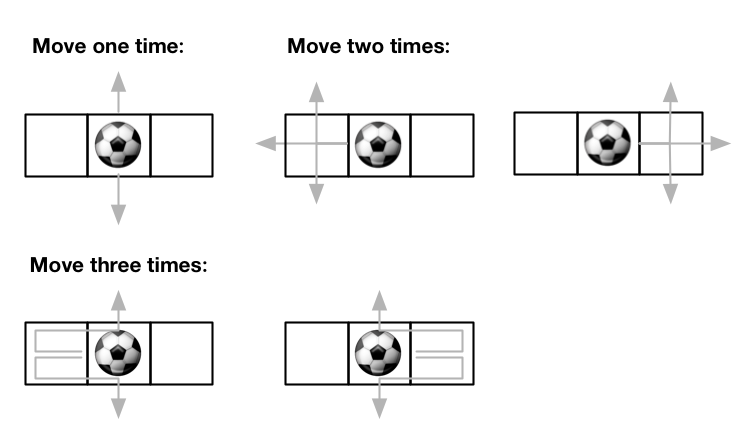
Input: m = 1, n = 3, maxMove = 3, startRow = 0, startColumn = 1
Output: 12
3. Constraints
1 <= m, n <= 50
0 <= maxMove <= 50
0 <= startRow < m
0 <= startColumn < n
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/out-of-boundary-paths 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
dp
class Solution {
public int findPaths(int m, int n, int maxMove, int startRow, int startColumn) {
final int kMod = 1000000007;
int[][][] dp = new int[maxMove + 1][m][n];
int[] dirs = {-1, 0, 1, 0, -1};
for (int s = 1; s <= maxMove; s++) {
for (int y = 0; y < m; y++) {
for (int x = 0; x < n; x++) {
for (int d = 0; d < 4; d++) {
int tx = x + dirs[d];
int ty = y + dirs[d + 1];
if (tx < 0 || ty < 0 || tx >= n || ty >= m) {
dp[s][y][x] += 1;
} else {
dp[s][y][x] = (dp[s][y][x] + dp[s - 1][ty][tx]) % kMod;
}
}
}
}
}
return dp[maxMove][startRow][startColumn];
}
}